Often developers are confused between the JavaScript Equality Operators. In this article we will look How many types of Equality Comparison Operator are there and What is the difference between Equality Comparisons == and === in JavaScript?
Types of Equality Comparisons in JavaScript
JavaScript provides three different value-comparison operations:
- strict equality (or “triple equals” or “identity”) using ===,
- loose equality (“double equals”) using ==,
- and
Object.is
(new in ECMAScript 2015).
Difference between Equality Comparisons == and === in JavaScript
Loose Equality(==)
The double equals will perform a type conversion when comparing two things in JavaScript. This is often considered as the slowest in the JavaScript when you are comparing the two different object types. Many developers do not recommend to use this approach for the comparison.
Strict Equality(===)
The Triple equals will behave almost like == operator but the only difference is it will not perform any type conversion, It just compares two objects and if the type is same it returns true else it will returns false.
The strict equality operator is much faster than loose equality as it doesn’t have to do any type conversion. It is always recommended to use === when you are not sure about the object types.
Object.is
Object.is
will behave the same way as triple equals, but with special handling for NaN
and -0
and +0
so that the last two are not said to be the same, while Object.is(NaN, NaN)
will be true
.
Examples of == vs ===
var num = 0; var obj = new String("0"); var str = "0"; var b = false; console.log(num === num); // true console.log(num == num); // true console.log(obj === obj); // true console.log(obj == obj); // true console.log(str === str); // true console.log(str == str); // true console.log(num === obj); // false console.log(num == obj); // true console.log(num === str); // false console.log(num == str); // true console.log(obj === str); // false console.log(obj == str); // true console.log(null === undefined); // false console.log(null == undefined); // true console.log(obj === null); // false console.log(obj == null);// false console.log(obj === undefined); // false console.log(obj == undefined); // false
Also, check out the very good example provided by the Mozilla Developer Network.
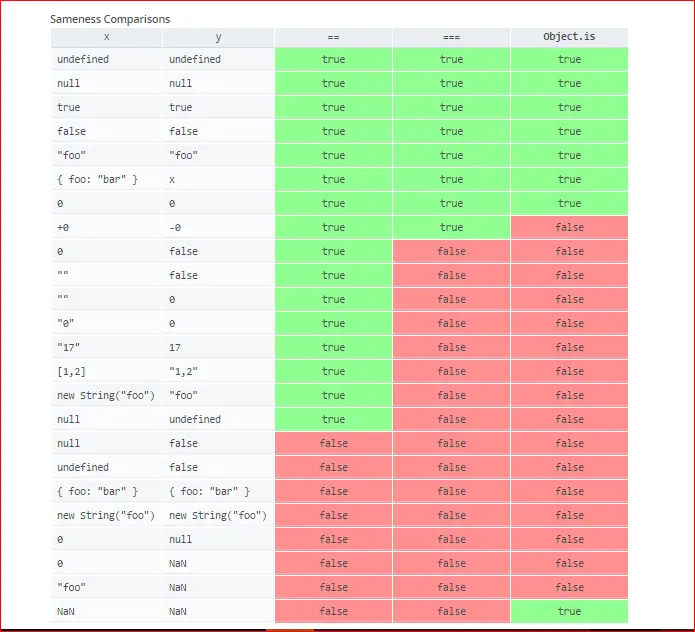